
Shows how to build & debug Rust in VS Code.In this case, demonstrated from linux. Rust Extension Pack is a collection of extensions that can help you write and test Rust applications in Visual Studio Code.
I recently wanted to debug a Rust program on Windows that I had written, and was struggling with how to get it to work. Since there was quite a lot of hoops to jump through to get it to work, I though I should share it with other people as well. I've written this blog post from memory and I'm far from an expert in LLVM or Rust, so if you see something strange here then let me know.
The drawback of this approach is that you can't debug applications built using the MSVC toolchain. If you know how to do this, please tell me. I would really appreciate it.
UPDATE 2018-02-02:
As Stuart Dootson pointed out to me, there's a guide on how to debug applications using the MSVC toolchain here. I haven't tried this out myself, but it looks promising. Thanks Stuart!
1. Install LLVM
First we need to install LLVM which is a compiler infrastructure. Part of LLVM is LLDB which is the debugger we're going to use. The latest version of LLVM doesn't include Python support for LLDB which is required for the VSCode extension, so we need to download a custom built version and install that.
2. Install Python
We now need to install Python v3.5 (Not v3.6).
https://www.python.org/downloads/release/python-354/
If you installed the x64 version of LLVM, make sure that you install the x64 version of Python as well, otherwise you will encounter problems later on.
3. Make sure it works
Make sure that lldb can use scripting. If this doesn't work, make sure that both LLVM and Python is on PATH.
Write exit()
to close the Python interpreter and then CTRL+C
to break out of LLDB.
4. Install the VSCode extension
We now need to install the vscode-lldb
extension for Visual Studio Code. This is what makes it possible to debug our Rust code from Visual Studio Code.
https://marketplace.visualstudio.com/items?itemName=vadimcn.vscode-lldb
5. Install the Rust GNU toolchain
Since LLDB doesn't work with the msvc compiler, we need to install the Rust GNU toolschain and use that one. This have some drawbacks though such as we won't be able to intop with software produced by Visual Studio.
6. Set the active toolchain
We now need to set the Rust GNU toolchain as the active toolchain. We do this by using the rustup
command again.
We should now verify what our toolchain configuration look like by using rustup show
.
7. Create a project
Create a new project called hello_world
.
Open Visual Studio Code and go to ./src/main.rs
and place a breakpoint.
8. Edit launch.json
Press F5 to run the project. A selection box will appear that will ask you what environment you want to use. Choose LLDB Debugger
here. This will open up the launch.json
file, and here we need to tell Visual Studio Code how to launch our project. Edit the file so it looks similar to this:
9. Profit
Press F5 again. You should now be debugging your code.
-->In this article, you use Visual Studio Code to create a custom handler function that responds to HTTP requests. After testing the code locally, you deploy it to the serverless environment of Azure Functions.
Custom handlers can be used to create functions in any language or runtime by running an HTTP server process. This article supports both Go and Rust.
Completing this quickstart incurs a small cost of a few USD cents or less in your Azure account.
Configure your environment
Before you get started, make sure you have the following requirements in place:
An Azure account with an active subscription. Create an account for free.
Visual Studio Code on one of the supported platforms.
The Azure Functions extension for Visual Studio Code.
The Azure Functions Core Tools version 3.x. Use the
func --version
command to check that it is correctly installed.Go, latest version recommended. Use the
go version
command to check your version.
An Azure account with an active subscription. Create an account for free.
Visual Studio Code on one of the supported platforms.
The Azure Functions extension for Visual Studio Code.
The Azure Functions Core Tools version 3.x. Use the
func --version
command to check that it is correctly installed.Rust toolchain using rustup. Use the
rustc --version
command to check your version.
Create your local project
In this section, you use Visual Studio Code to create a local Azure Functions custom handlers project. Later in this article, you'll publish your function code to Azure.
Choose the Azure icon in the Activity bar, then in the Azure: Functions area, select the Create new project... icon.
Choose a directory location for your project workspace and choose Select.
Note
These steps were designed to be completed outside of a workspace. In this case, do not select a project folder that is part of a workspace.
Provide the following information at the prompts:
Select a language for your function project: Choose
Custom
.Select a template for your project's first function: Choose
HTTP trigger
.Provide a function name: Type
HttpExample
.Authorization level: Choose
Anonymous
, which enables anyone to call your function endpoint. To learn about authorization level, see Authorization keys.Select how you would like to open your project: Choose
Add to workspace
.
Using this information, Visual Studio Code generates an Azure Functions project with an HTTP trigger function. You can view the local project files in the Explorer. To learn more about files that are created, see Generated project files.
Create and build your function
The function.json file in the HttpExample folder declares an HTTP trigger function. You complete the function by adding a handler and compiling it into an executable.
Press Ctrl + N (Cmd + N on macOS) to create a new file. Save it as handler.go in the function app root (in the same folder as host.json).
In handler.go, add the following code and save the file. This is your Go custom handler.
Press Ctrl + Shift + ` or select New Terminal from the Terminal menu to open a new integrated terminal in VS Code.
Compile your custom handler using the following command. An executable file named
handler
(handler.exe
on Windows) is output in the function app root folder.
Press Ctrl + Shift + ` or select New Terminal from the Terminal menu to open a new integrated terminal in VS Code.
In the function app root (the same folder as host.json), initialize a Rust project named
handler
.In Cargo.toml, add the following dependencies necessary to complete this quickstart. The example uses the warp web server framework.
In src/main.rs, add the following code and save the file. This is your Rust custom handler.
Compile a binary for your custom handler. An executable file named
handler
(handler.exe
on Windows) is output in the function app root folder.
Configure your function app
The function host needs to be configured to run your custom handler binary when it starts.
Open host.json.
In the
customHandler.description
section, set the value ofdefaultExecutablePath
tohandler
(on Windows, set it tohandler.exe
).In the
customHandler
section, add a property namedenableForwardingHttpRequest
and set its value totrue
. For functions consisting of only an HTTP trigger, this setting simplifies programming by allow you to work with a typical HTTP request instead of the custom handler request payload.Confirm the
customHandler
section looks like this example. Save the file.
The function app is configured to start your custom handler executable.
Run the function locally
You can run this project on your local development computer before you publish to Azure.

In the integrated terminal, start the function app using Azure Functions Core Tools.
With Core Tools running, navigate to the following URL to execute a GET request, which includes
?name=Functions
query string.http://localhost:7071/api/HttpExample?name=Functions
A response is returned, which looks like the following in a browser:
Information about the request is shown in Terminal panel.
Press Ctrl + C to stop Core Tools.
After you've verified that the function runs correctly on your local computer, it's time to use Visual Studio Code to publish the project directly to Azure.
Sign in to Azure
Before you can publish your app, you must sign in to Azure.
If you aren't already signed in, choose the Azure icon in the Activity bar, then in the Azure: Functions area, choose Sign in to Azure.... If you don't already have one, you can Create a free Azure account. Students can create a free Azure account for Students.
If you're already signed in, go to the next section.
When prompted in the browser, choose your Azure account and sign in using your Azure account credentials.
After you've successfully signed in, you can close the new browser window. The subscriptions that belong to your Azure account are displayed in the Side bar.
Compile the custom handler for Azure
In this section, you publish your project to Azure in a function app running Linux. In most cases, you must recompile your binary and adjust your configuration to match the target platform before publishing it to Azure.
In the integrated terminal, compile the handler to Linux/x64. A binary named
handler
is created in the function app root.
Create a file at .cargo/config. Add the following contents and save the file.
In the integrated terminal, compile the handler to Linux/x64. A binary named
handler
is created. Copy it to the function app root.If you are using Windows, change the
defaultExecutablePath
in host.json fromhandler.exe
tohandler
. This instructs the function app to run the Linux binary.Add the following line to the .funcignore file:
This prevents publishing the contents of the target folder.
Publish the project to Azure
In this section, you create a function app and related resources in your Azure subscription and then deploy your code.
Important
Publishing to an existing function app overwrites the content of that app in Azure.
Choose the Azure icon in the Activity bar, then in the Azure: Functions area, choose the Deploy to function app... button.
Provide the following information at the prompts:
Select folder: Choose a folder from your workspace or browse to one that contains your function app. You won't see this if you already have a valid function app opened.
Select subscription: Choose the subscription to use. You won't see this if you only have one subscription.
Select Function App in Azure: Choose
+ Create new Function App (advanced)
.Important
The
advanced
option lets you choose the specific operating system on which your function app runs in Azure, which in this case is Linux.Enter a globally unique name for the function app: Type a name that is valid in a URL path. The name you type is validated to make sure that it's unique in Azure Functions.
Select a runtime stack: Choose
Custom Handler
.Select an OS: Choose
Linux
.Select a hosting plan: Choose
Consumption
.Select a resource group: Choose
+ Create new resource group
. Enter a name for the resource group. This name must be unique within your Azure subscription. You can use the name suggested in the prompt.Select a storage account: Choose
+ Create new storage account
. This name must be globally unique within Azure. You can use the name suggested in the prompt.Select an Application Insights resource: Choose
+ Create Application Insights resource
. This name must be globally unique within Azure. You can use the name suggested in the prompt.Select a location for new resources: For better performance, choose a region near you.The extension shows the status of individual resources as they are being created in Azure in the notification area.
When completed, the following Azure resources are created in your subscription:
- A resource group, which is a logical container for related resources.
- A standard Azure Storage account, which maintains state and other information about your projects.
- A consumption plan, which defines the underlying host for your serverless function app.
- A function app, which provides the environment for executing your function code. A function app lets you group functions as a logical unit for easier management, deployment, and sharing of resources within the same hosting plan.
- An Application Insights instance connected to the function app, which tracks usage of your serverless function.
A notification is displayed after your function app is created and the deployment package is applied.
Select View Output in this notification to view the creation and deployment results, including the Azure resources that you created. If you miss the notification, select the bell icon in the lower right corner to see it again.
Run the function in Azure
Back in the Azure: Functions area in the side bar, expand your subscription, your new function app, and Functions. Right-click (Windows) or Ctrl - click (macOS) the
HttpExample
function and choose Execute Function Now....In Enter request body you see the request message body value of
{ 'name': 'Azure' }
. Press Enter to send this request message to your function.When the function executes in Azure and returns a response, a notification is raised in Visual Studio Code.
Clean up resources
When you continue to the next step and add an Azure Storage queue binding to your function, you'll need to keep all your resources in place to build on what you've already done.
Otherwise, you can use the following steps to delete the function app and its related resources to avoid incurring any further costs.
Rust Visual Studio 2017
In Visual Studio Code, press F1 to open the command palette. In the command palette, search for and select
Azure Functions: Open in portal
.Choose your function app, and press Enter. The function app page opens in the Azure portal.
In the Overview tab, select the named link next to Resource group.
In the Resource group page, review the list of included resources, and verify that they are the ones you want to delete.
Select Delete resource group, and follow the instructions.
Deletion may take a couple of minutes. When it's done, a notification appears for a few seconds. You can also select the bell icon at the top of the page to view the notification.
Rust Visual Studio
To learn more about Functions costs, see Estimating Consumption plan costs.
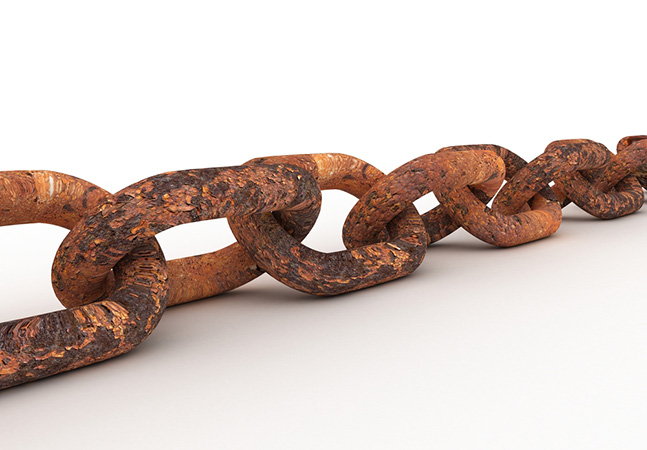
Visual Studio Code Rust Support
Next steps
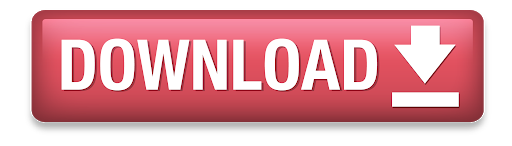